
In this tutorial, we are going to create a sample application on the recommended MVVM Architecture Pattern and you will notice how it improves overall code quality, maintenance and debugging of our app.
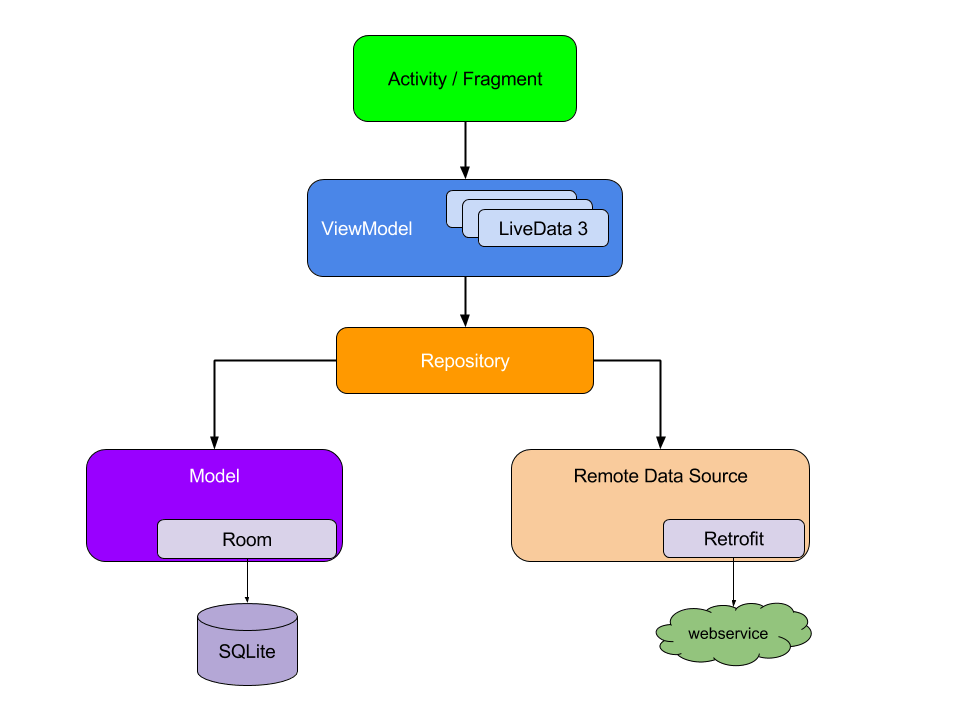
The above figure shows the MVVM design pattern. Android App Development Company India will build all these components step by step and notice the dependency of one component on the other. Check the docs to know more about the design pattern. As you can see the UI components are kept away from the business logic of app. The activity will use the ViewModel to get the data where the ViewModel will use the Repository to get the data which contains data from different data sources.
Our app will query to the Food2Fork API using a SearchView and display the corresponding recipes in a RecyclerView. So head there and generate an API Key.
Start by creating a new Android Studio project name it anything of your choice.
Adding the Dependencies
Add the following dependencies to your build.gradle file -
(Check the latest version from the respective official docs.)
Note here we have added the ViewModel and LiveData dependencies.
Adding Internet Permission
Add the required INTERNET permission in the Manifest.xml so we can make network calls.
Now, create a package called utils and add a new ConstantsM class in it.
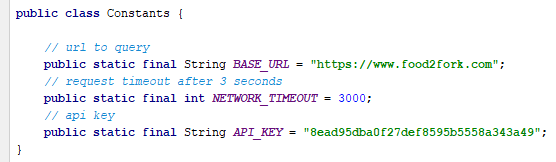
This class is consists of our URL, API key and a network timeout of 3 seconds.
Next, create a new package network which will hold all of our network logic. Inside this, we will add three classes. First one is an interface RecipeApi -
We create a method searchRecipe which returns a Retrofit Call object. The @GET annotation denotes what kind of HTTP request we are going to make.
Now create a class named ServiceGenerator which acts as a controller for our Api Client -
We add the Food2Fork API’s URL that we instantiated in Constants class. In the addConverterFactory() method, we add the GsonConverterFactory for serialization and deserialization of objects and then pass all these to our Retrofit instance.
The third class is our ApiClient so create it and leave it blank for now.
Model JSON Response
Let’s now take a look at the JSON response we are going to parse. You can check the Food2Fork docs for a sample response. Our URL, in this case, is
https://www.food2fork.com/api/search?key=YOUR_API_KEY&q=cake&page=1
The response you get is -
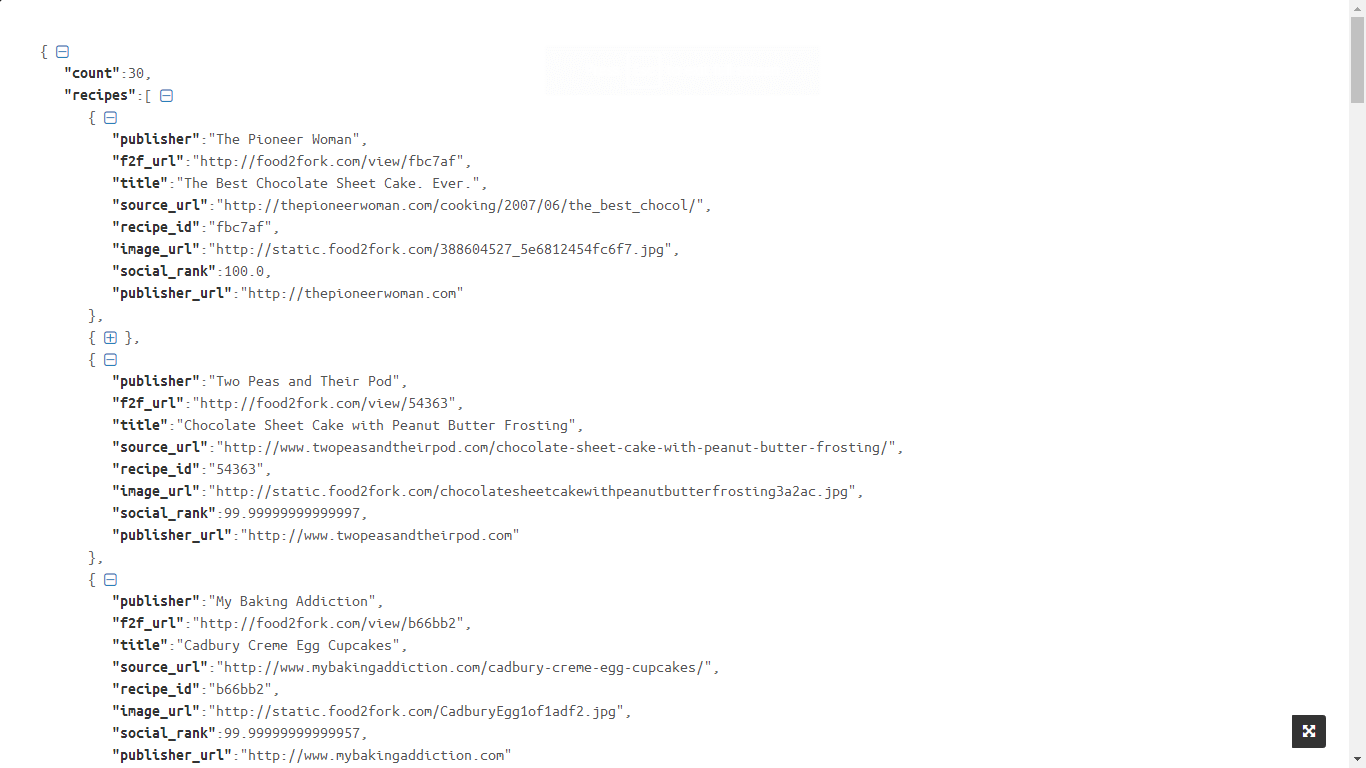
So now we will model this JSON Resopnse. So, we will create a new package called model, and we will add two classes here -
-
FoodResponse
public class FoodResponse { @SerializedName("recipes") @Expose private List recipes; public List getRecipes() { return recipes; } } -
Recipe -
This is a simple data class reflecting the JSON
public class Recipe{ private String title, publisher, image_url; public Recipe(String title, String publisher, String image_url) { this.title = title; this.publisher = publisher; this.image_url = image_url; } public Recipe() { } public String getTitle() { return title; } public String getPublisher() { return publisher; } public String getImage_url() { return image_url; } }
Now we will create an Executor Class. So, create a new class called AppExecutors -
This class will fire the background threads when we need them, as all of the Retrofit requests need to be done on the background thread. The class uses the Singleton pattern, which is a software design pattern that restricts instantiation of a class/object to a single one. We are using the ScheduledExecutorService as it allows extra functionalities compared to the classic Executor, and can run after a time delay. Then we create a pool of thread, 3 threads to do all the work required in the app. Later we will use this networkIO object to set a timeout.
We will use the schedule() method of ScheduledExecutorService to set a network timeout and can cancel the request.
Now let’s head back to our ApiClient class. Later our repository will observe this data source and send it to the ViewModel.
-
> mRecipes;
private RetrieveRecipesRunnable mRetrieveRecipesRunnable;
public static ApiClient getInstance(){
if(instance == null){
instance = new ApiClient();
}
return instance;
}
private ApiClient() {
mRecipes = new MutableLiveData<>();
}
public LiveData
-
> getRecipes(){
return mRecipes;
}
public void searchRecipesApi(String query, int pageNumber){
if(mRetrieveRecipesRunnable != null){
mRetrieveRecipesRunnable = null;
}
mRetrieveRecipesRunnable = new RetrieveRecipesRunnable(query, pageNumber);
final Future handler = AppExecutors.getInstance().networkIO().submit(mRetrieveRecipesRunnable);
// Set a timeout for the data refresh
AppExecutors.getInstance().networkIO().schedule(new Runnable() {
@Override
public void run() {
// let the user know it timed out
handler.cancel(true);
}
}, NETWORK_TIMEOUT, TimeUnit.MILLISECONDS);
}
private class RetrieveRecipesRunnable implements Runnable{
private String query;
private int pageNumber;
private boolean cancelRequest;
private RetrieveRecipesRunnable(String query, int pageNumber) {
this.query = query;
this.pageNumber = pageNumber;
cancelRequest = false;
}
@Override
public void run() {
try {
Response response = getRecipes(query, pageNumber).execute();
if(cancelRequest){
return;
}
if(response.code() == 200){
List
This is a lot of code, so let’s understand it. This class is also built on the Singleton pattern. Next, we instantiate a MutableLiveData object in the constructor, and have an associated getter method.
Let’s talk about LiveData a bit. LiveData follows the Observer pattern and this Observer is notified whenever lifecycle changes.This leads to a clean code structure as whenever your data updates it is reflected in the UI. Moreover when an activity is destroyed the observer associated with it gets destroyed too, preventing memory leaks.You also don’t need to explicitly configure lifecycle events as while observing LiveData is aware of lifecycle events, example you don’t need to manage the configure changes!
Also MutableLiveData is just a subclass of LiveData and its value can be changed contrast to the LiveData, and LiveData can only be observed.
Now since we are going to make request in the ApiClient, we make new method searchRecipesApi. Inside this we create a Future object which is going to make the call and set a timeout as well. In the submit method we pass our own Runnable class implementation inside of which we are calling Retrofit’s execute() method, and posting the response to our LiveData object. Also inside of our searchRecipesApi method we call schedule() method which takes three parameters, viz, a Runnable, time duration and the Time Unit. Inside this we cancel our Future object denothing the request timeout.
Next create a new package called as repositories and add class RecipeRepository to it -
-
> getRecipes(){
return mApiClient.getRecipes();
}
public void searchRecipesApi(String query, int pageNumber){
if(pageNumber == 0){
pageNumber = 1;
}
mQuery = query;
mPageNumber = pageNumber;
mApiClient.searchRecipesApi(query, pageNumber);
}
public void searchNextPage(){
searchRecipesApi(mQuery,mPageNumber+1);
}
}
It is also based on singleton pattern and as seen in the diagram further takes our LiveData away from the view, as shown in the MVVM diagram above. Acting as an intermediary between the ViewModel and the data source. So the implementation here is pretty straightforward and we create an ApiClient object and then we search recipes by calling the searchRecipesApi method of our ApiClient.
Now before jumping on anything else, let’s take a second to create the layouts for our app. So, create a new Layout resource file layout_recipe_list_item -
The layout tells what each item inside of our RecyclerView should be displayed as. We have refactored the absolute sizes in a dimens xml file which contains following attributes -
In the layout of MainActivity we will add a RecyclerVew and a SearchView, using CoordinatorLayout as parent. Also set App theme as Theme.AppCompat.Light.NoActionBar in res/styles.xml.
Now create a new package called adapter, and as you might have guessed it will have logic have a viewholder and a RecyclerViewAdapter. Create a new class CustomAdapter -
The CustomViewHolder inner class contains the views which are needed to be bind to the adapter. We also use Glide library to load the image into our image view and if you have been developing apps this would be very familiar to you.
Now create a new package viewmodel and inside of it create a new class MainActivityViewModel -
-
> getRecipes() {
return mRecipeRepository.getRecipes();
}
public Boolean IsViewingRecipes() {
return mIsViewingRecipes;
}
public void setIsQueryingRecipes(boolean mIsQueryingRecipes) {
this.mIsQueryingRecipes = mIsQueryingRecipes;
}
public void searchRecipesApi(String query, int pageNumber){
mIsViewingRecipes = true;
mRecipeRepository.searchRecipesApi(query, pageNumber);
mIsQueryingRecipes = true;
}
public void searchNextPage(){
if (!mIsQueryingRecipes && mIsViewingRecipes){
mRecipeRepository.searchNextPage();
}
}
}
The ViewModel class is responsible for passing the data to our Activity from the Repository. Main function of this class is to avoid the loss of data on configuration changes. We extend the ViewModel class and create an instance variable of repository class, and use it to get the data.
You can also use member variables like mIsQueryingRecipes and mIsViewingRecipes to know if the actions on the UI which is a great way for architecturing your app.
Now we are ready to go to our MainActivity and implement this ViewModel. In our activity we get the viewmodel from the framework utility class called ViewModelProviders which takes an activity instance.
Next we use our ViewModel object to call the getRecipes method which returns a LiveData and observe this LiveData and pass a context and Observer as argument. So, we will use its onChanged() method which triggers when a change is made to our LiveData object.
Here is the implementation -
-
>() {
@Override
public void onChanged(@Nullable List
Note how we are using search view to query the data. Run the app and enter a query, example `Cake`, and you should see a list of Cake recipes and can match it from the Food2Fork API. But here is a catch. If you run the app on API level 28, Glide won’t be able to load images. This is as network security configuration options have changed and you can’t simply load resources from HTTP requests though HTTPS would work fine.
So, create a new directory xml inside the res folder. Now create a new Network configuration file network_security_config.xml -
After this go to Manifest and reference this file by adding the tag and run app again -
You have successfully implemented MVVM architecture