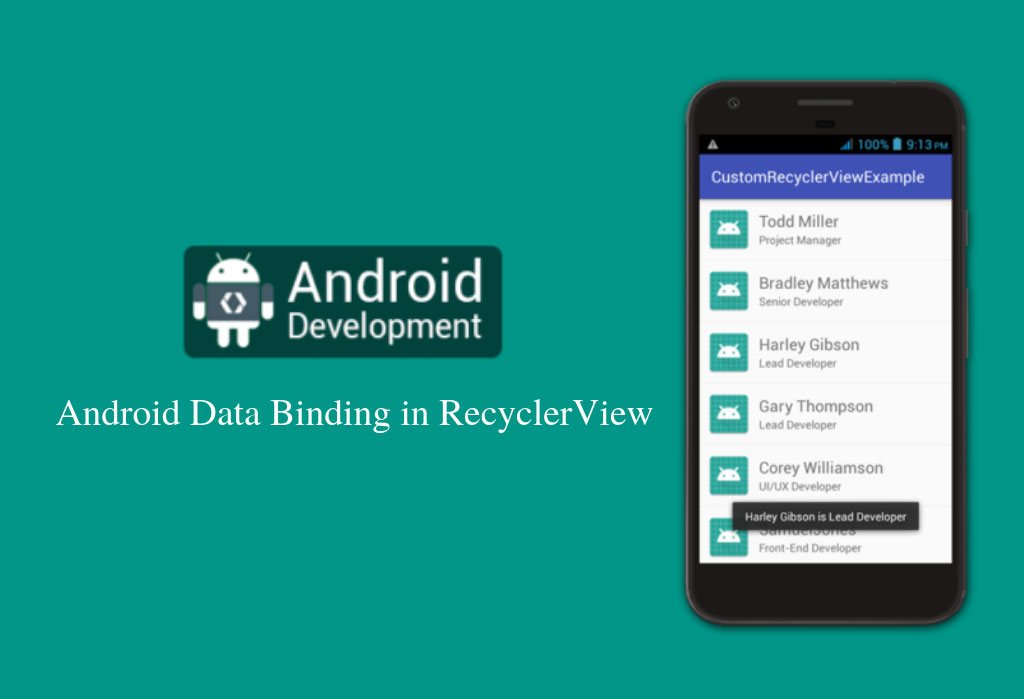
Databinding in android give you the easiest and much powerful way to connect UI elements of your application’s layout with business logic by using declarative layouts which minimizes your effortsof writing code and update the layouts programmatically without manual interference. It also integrates Java/Kotlin code with xml layouts and taking good care of views and keep them upgraded without any interruption.Not only this, it also raise your app’s performance and making it more flexible by preventing it from crashing.
Connecting the components of layout files with data objects helps you avoiding many UI framework calls in your activities and make them simplify and easier to maintain. Android databinding support library is used to support this feature which is compatible with all the latest android versions and creates thebinding classes that are required to tie layout’s views with your data objects.
Project Setup
To implement a databinding in your application you first need to enable this feature in app/build.gradle file of your project and a layout files in which you want to use.
EnabeDatabinding in app/build.gradle
In build.gradle file of your project under app, enable the databinding by adding the following code snippet and sync the project.
Build.gradle
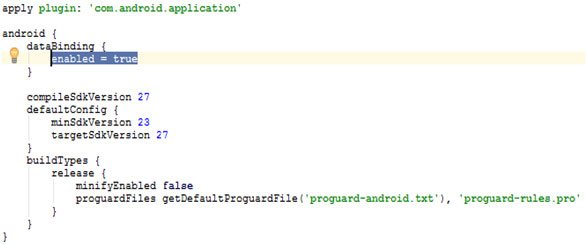
EnableBindingin a layout File
You have to make some changes in a layout files in which you want to enable databinding. Databinding layouts are slightly different and start the layout file with the <layout> root tag proceeding by a data element and a view root elements.The data element will be used to represent the data which is ready to use for binding and the view element represents the root hierarchy which would be in the layout files other than binding layouts. See the below example in which data model object variable is defined under <data>tag using variable element.
Binding Expressions
Binding expressions are actually used in expression language to write the expressions that allows variables to make connection with the views in the layout files.
The @{} symbol in theassignment expressions is used to make connections between attribute of views and the property of data model object. Generally, we use setText() methods to set data in a textView but instead of using this method and calling it manually for each property we are assigning text to a widgets directly in a layout file.
Observable data objects
Android data binding support library remove all your worries by providing classes and methods which will automatically detects if any changes occurs in data source and allowing you to handle them by making the variables or their properties observable. You can make your fields, objects or collection observable with the help of this library.
Binding classes
The data binding library auto generatesbinding classes on the bases ofeach binding layout file and grasp all the bindings from the layout properties. By default, the name of the binding class will be set according to the name of the layout file and convert it into the pascal case by adding the Binding suffix at the end of the class name. Here, Android app development India are explain an example, if the layout file name is activity_main.xml then the binding class name will be ActivityMainBinding.
Implementation
In this tutorial I am going to display phone contacts list by implementing data binding using recycler view. For recycler view, you must need to add the following dependency in your build.gradle file of your project under appmodule.
Build.gradle
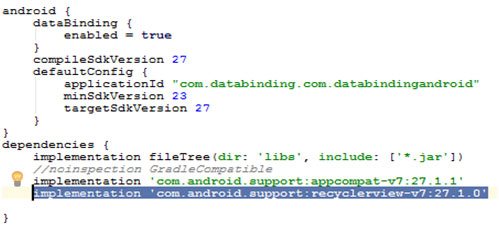
Adding resource to your project:
dimens.xml
Binding Views element with data model objects
- Represents a single row item in a recycler view.Add the below code in contacts_row_item.xml file.
- @{contacts.name},@{contacts.name}are the view properties in textView that are used to make the connection with data objects in Contacts.java class and @{contacts.getImageUrl()} is used to bind ImageView property with the method in Contacts.java file.
contacts_row_item.xml
Create a java class Contacts.java which is data object model class inside a package name model and add the below code snippet in it. This class has three properties that are binded to the views of the layout file contacts_row_item.xml.
Contacts.java
Create a CustomImageBinding.java class and use BindAdapters to bind the data object with the image view in thelayout file using @BindingAdapter({"android:src"})
CustomImageAdapter.java
Bind the layout view of the contacts_row_item.xml file with binder object and get access to it.
ContactsViewHolder.java
Create an adapter class ContactsAdapter.java and add the below code snippet.
ContactsAdapter.java
MainActivity.java
Application at Runtime:
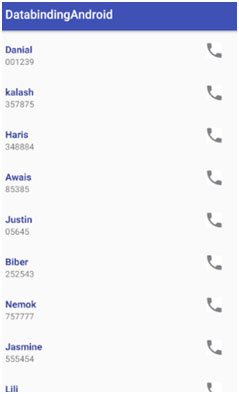