Progress bar or spinner loaders are used in applications to load the data on screens whenever application gets the data from a network call. These spinners are in horizontal or circular styles displays on screens to load data but now lets have some change in your android applications to make them more interesting and impressive by using cool shimmer animation in your application’s views which makes your front end design more attractive and appealing.
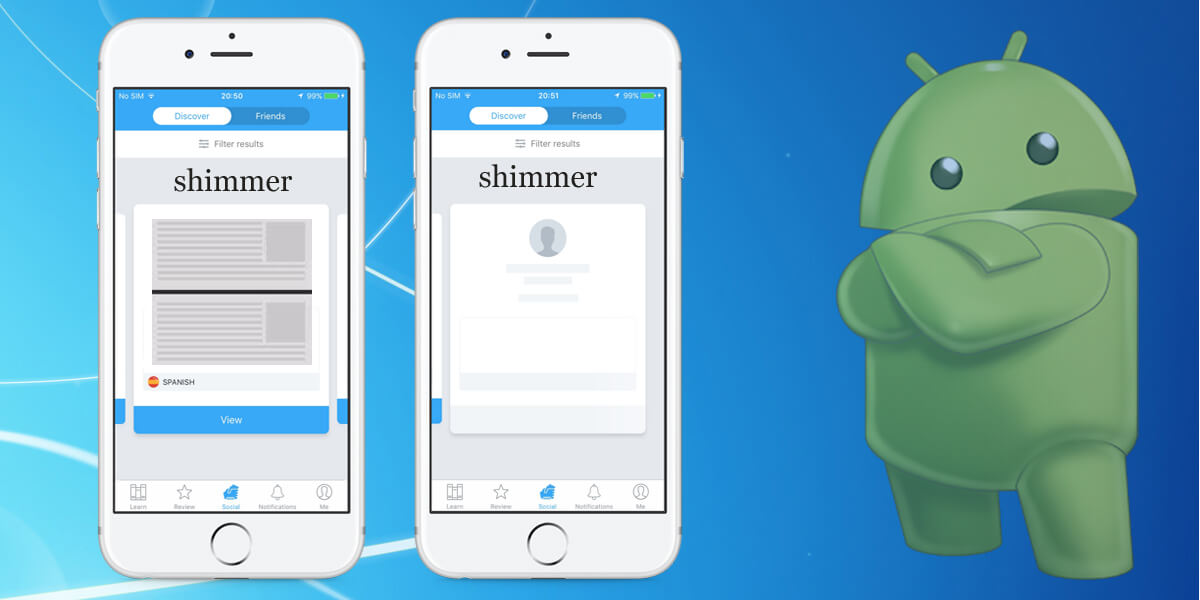
For this you have to use an open source android shimmer library which was intimately developed by facebook and not only implemented by facebook but by linkedin and udemy as well. This feature is used by Facebook in a way like displaying status when loading the news feed, wallpaper settings and opening notifications.
Simmer is an android layout which nest any view inside it using a “ShimmerFrameLayout” tag.You can specify the values for the layout either on the tag(using custom attributes) or programmatically in your code, and generate an animation on the fly.In order to get this services don’t forget to use the shimmer dependency in your application.
In the given example below I have developed a simple android project in which a shimmer animation is applied to a list view data. Shimmer will start as the application get started and will stop on button click with displaying the data on a listview.You can obtain shimmer effects in any view in your application wherever you want with the help of ShimmerFrameLayout.
Here we go for android project…!
1. Project creation:
- Open the development environment android studio click on File ->New->New Project->then select the basic activity from layouts.
- In order to use shimmer effects in your application’s layout , you have to add the shimmer dependency in your project’s build.gradle(module level) file and rebuild the project.
build.gradle
- I have created an xml file named as activity_main.xml with constraint layout and add the ShimmerFrameLayout inside it.Add the below code in activity_main.xml file of your project’s resources folder.
activity_main.xml
-
In the file below I have created two custom views for listview. By default listview provide one view but I needed two in my project so I have created custom listview in my project.
Make another layout file placeholder.xml in a layout folder to add views for your shimmer layout. I have made two views in this file and used this layout in activity_main.xml file using <include layout="@layout/placeholder" attribute/>
placeholder.xml
- Add the below code in dimen file of our project’s resources.
dimens.xml
- Add the below code in Strings.xml file of your project resources.
Strings.xml
- Below is the code snippet to get the Shimmer effect.
In this file I have created string type array in which I have stored string data to use it later in the listview.
- Initialize the shimmer in java file with the shimmer view in layout file .
ShimmerFrameLayout shimmer_layout=(ShimmerFrameLayout) findViewById(R.id.shimmer_view);
- To start the shimmer animation, call startShimmerAnimation() function of the android’s ShimmerFrameLayout class.
- To stop the shimmer animation, call stopShimmerAnimation() function of the android’s ShimmerFrameLayout class.
- Initialize all the layout views in MainActivity.java file
shimmer_layout=(ShimmerFrameLayout) findViewById(R.id.shimmer_view);
listview=(ListView)findViewById(R.id.list);
btn_load=(Button) findViewById(R.id.button);
- Initially I have set listview’s visibility to Gone and start the shimmer animation.As I click on the button the shimmer will stop and the data in a listview will be displayed on the screen
listview.setVisibility(View.GONE);
shimmer_layout.startShimmerAnimation();
- load_data() function will be invoked on button click
btn_load.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
load_data();
}
});
- Get string array data from string.xml file of your project’s resources folder
res_names=getResources().getStringArray(R.array.Res_names);
Inside load_data() function shimmer animation will stop using stopShimmerAnimation() function and its visibility will be Gone on setVisibility(View.GONE) function.
ListAdapter will load the data in a listview with the help of setAdapter(customizedList) method.
Add the below full code snippet in MainActivity.java
MainActvity.java
Cutomized list class is used as a single cell oflistview.
customizedList.java
2. Running android Application
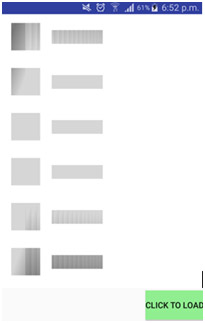
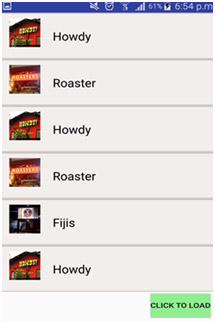
Shimmer effects on Custom views
Shimmer Attributes: Shimmer provides multiple custom attributes which you can command to change the appearance and pace of the shimmer animations.
- Shimmer angle The angle must be between -45 and 45.It defines the angle of the shimmer line. Default angle value is 20.
- Shimmer autoStart Default value for this feature is false. shimmer_auto_start function is used to start the animation automatically without calling startShimmerAnimation method.
- Shimmer color This attribute describe the color of shimmer line.The center color and the edge color would be the same with the decreased alpha value and the default value for this attribute is #A2878787
- Shimmer animation duration Defines the total time of the animation in miliseconds.its default values is 1500
- Reversed Shimmer Animation Its default value is false which means the animation will start from the left side of the view but if you want to reverse the process, just change the value from false to true, the animation will start from the right side of the view.
- Shimmer center color width The shimmer line gradient will starts with the transparent edge and move towards the center by attaining the color given with the shimmer color attribute.The width of center is not constant, it can be modified easily using the attribute shimmer_gradient_center_color_width. Its default value is 0.1
- Shimmer mask width Its default value is 0.5 which means the gradient initially cover only 0.5 part of the width and shimmer line width is half of the layout’s width at angle 0. So when 1 value is given to this attribute, the gradient will cover half of the layout's width.
In this tutorial, Android application development India are sharing its best experience about "ShimmerFrameLayout" and how it implements for android project.