There are many times when we need to hide soft keyboard (also called virtual keyboard) when probably there is a focus change, or on click of a button (say “Submit”), or any other action. There could be a case where you don’t want keyboard to pop when user enters an Activity.
You can use any one of the many snippets present all over the web, but after hitting build only you will notice that it was supposed to be called from Activity, or maybe it required a view to be passed. Hence, there is no one size fits all solution for such a simple requirement.
Android provides a class InputMethodManager which deals with current input method for an application.
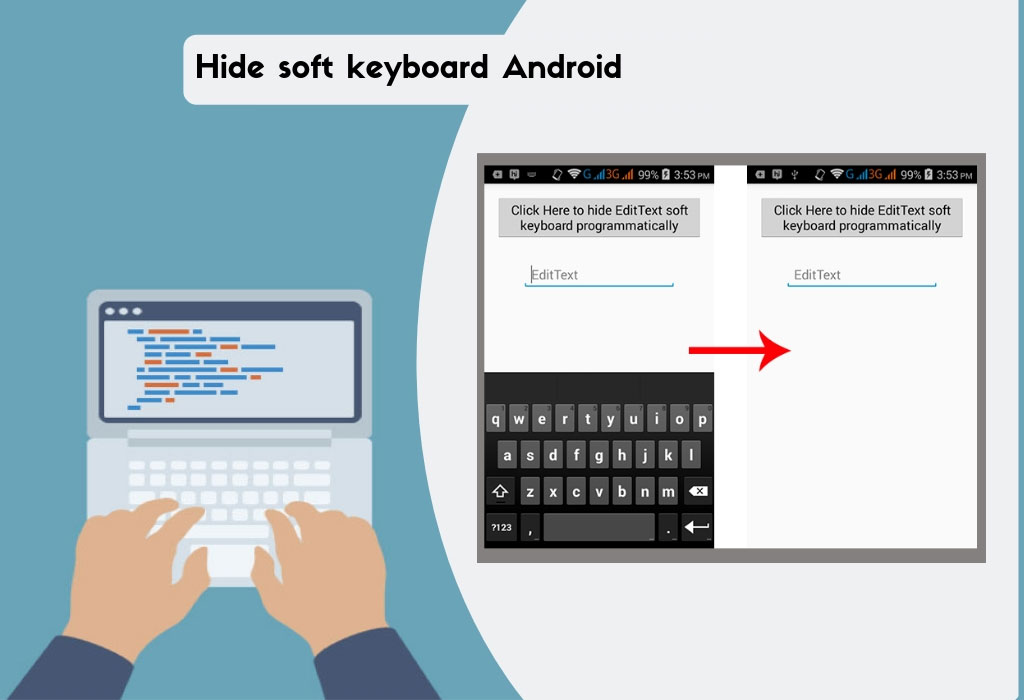
It would have been easier to directly call a method from InputMethodManager to hide or close the currently displayed keyboard, but this is where problem arises.
Context is required to get the hold of InputMethodManager instance. Getting a context is not a problem, but such methods are usually called from some sort of utility classes which don’t have context.
If it wasn’t frustrating enough to get the correct Context instance, InputMethodManager also requires the View from which keyboard needs to be hidden from. This is where novice as well as seasoned Android App Development Company feel cheated.
Here is a utility method which closes the soft keyboard BUT only when you call it from an Activity:
Sample call to closeKeyboard where MainActivity being your current Activity:
closeKeyboard(MainActivity.this);
Or:
closeKeyboard(this);
This method requires the current Activity instance in which you are trying to hide the soft keyboard.
The limitation of this utility method is that it requires you to call it from an Activity. This won’t work if calling from a Fragment. You may feel calling it away with Fragment’s parent Activity using getActivity() but it won’t work because while the fragment is displayed, parent Activity is not holding any focus.
To hide the soft keyboard from a Fragment, you can use following utility method:
This can be called by passing Context and the view from currently displayed screen where you want to hide the keyboard:
hideKeyboardFrom(getContext(), myEditView);
There could be cases when there is no focusable view in Fragment and you want to hide the keyboard due to some event or trigger.
Notice that we have changed the way we acquire the “View”.
If you choose the fragment as a parameter, you can update this method to use the Fragment instance to acquire the view.
Whenever an Activity is launched with a focusable view in it, SoftKeyboard is supposed to launch as soon as first focusable view is found because initial focus is assigned to this view. If you want to keep the keyboard hidden when an Activity is launched, you will need to update the “windowSoftInputMode” attribute in AndroidManifest.xml.
This restricts the automatic launch of keyboard when an Activity is launched but it won’t stop the keyboard from popping up when the focusable view is touched.
On the contrary, if the situation demands display of SoftKeyboard when an Activity is launched without having user to touch a focusable or editable view, you can modify the “windowSoftInputMode” attribute accordingly:
These are the few cases that deals with common keyboard scenarios. It’s a good practice to have precise control on keyboard according to use case rather than having Android decide as it can be really frustrating for user to manually close the keyboard when not required. It may lead to accidentally closing the current Activity or Fragment due to pressing back button. It hampers the current operation being performed. This may, unfortunately, result in user losing the interest in your application altogether.