Today, we are going to implement a simple android application for Android developers india to integrate User Registration using firebase authentication. The authentication process will be achieved by using users email addresses and passwords. This is made possible by the firebase authentication SDK that has methods that can be used to create and manage users who use their email addresses and passwords for signing in. It is also equipped with handling password reset emails. To achieve our agenda, we first need to integrate firebase in our android project.
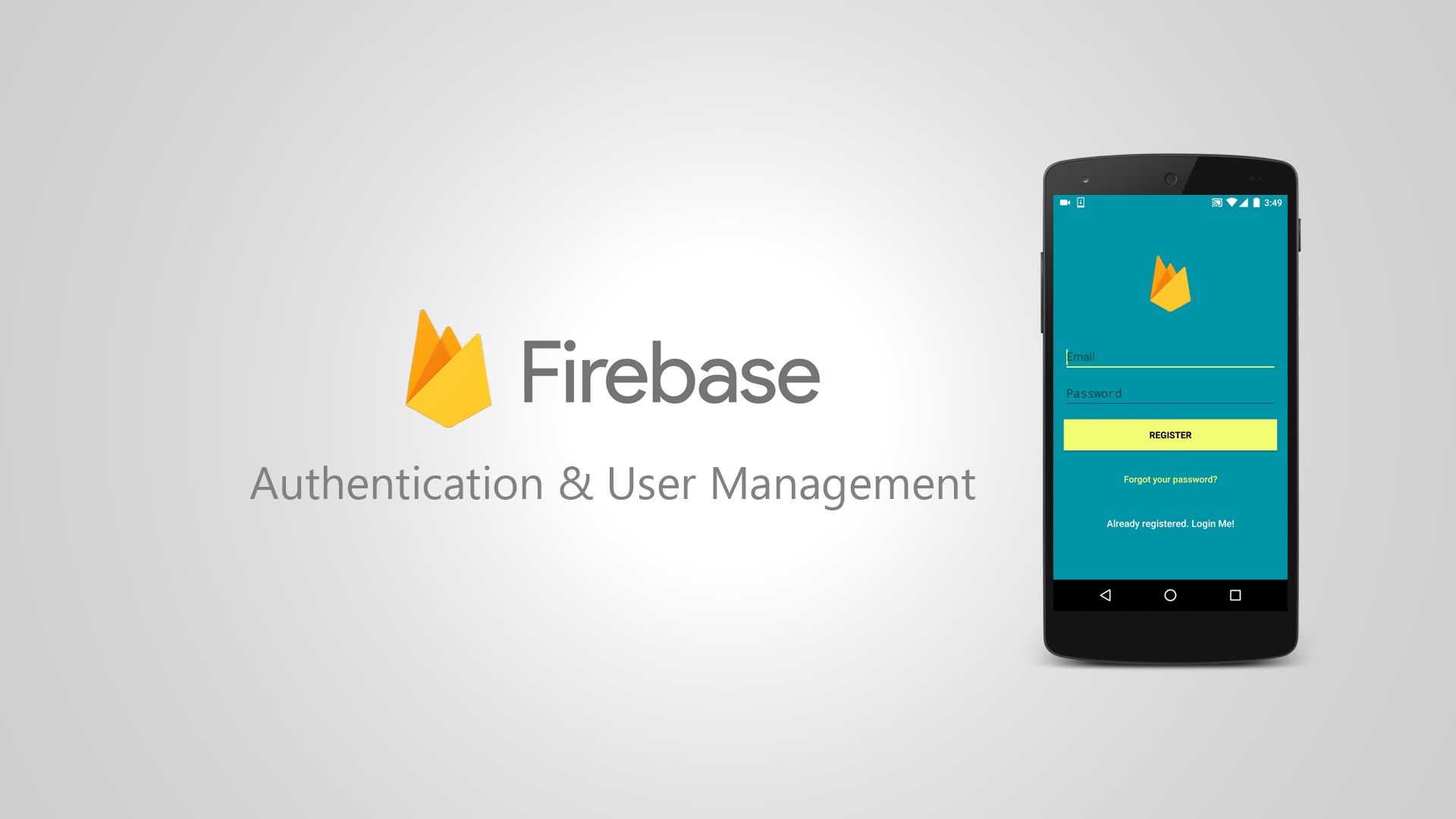
Integrating firebase into your android project:
1.Integrate a firebase manually
You’ll need to add a firebase manually if you are using android studio’s older version which is below 2.2 but if you are using the 2.2 or above versions you’ll simply need a firebase assistant to connect your android application with firebase. For doing this steps are discussed in a second heading.
First create android project
File ->New->New project.While filling the project details ,make sure to use the same package name in a firebase console. We are using com.firebaseandroid.com.firebaseandroidpackage.
You need to create a firebase project in a firebase console.Go to this link https://console.firebase.google.com/. You’ll see a “Welcome to firebase” window.
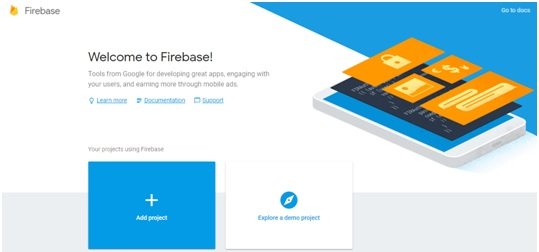
Steps:
1. Click “add project”
2. Use the same project name which you used while creating your android project. Our project name is “FirebaseAndroid”.

3. Select your country and click on a buttonCREATE PROJECT.
4. Click “Add Firebase to your Android app” and follow the steps in a from.
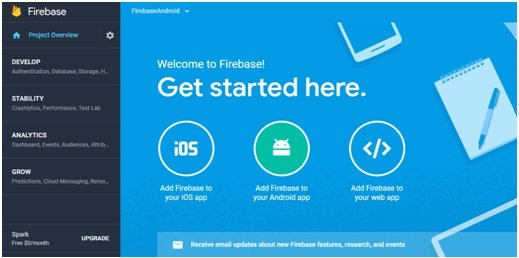
5. Make sure to give thesame package name that you used in your android project in which you are going to integrate the firebase. In our case package name is “com.firebaseandroid.com.firebaseandroid”.
6. Leave other two fields empty as they are optional and click the REGISTER APP button.
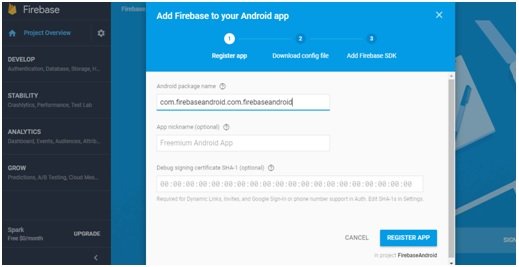
7. Download the google-services.jsonfile and place this file in your project’s module folder, typically/app. Your project won’t build without this file, So you can download this file at any time using this link https://support.google.com/firebase/answer/7015592

8. After clicking on a CONTINUE button , you’ll get the gradle instructions that you’ll need to put in your Android studio project’s files.
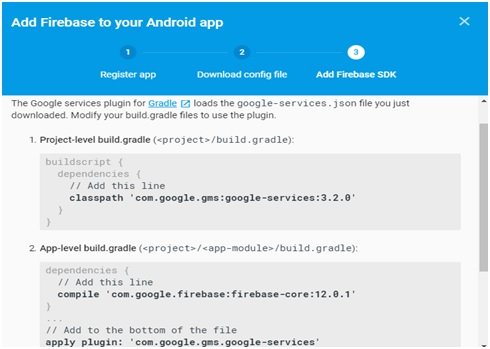
9. Add Firebase SDK
Open build.gradle file at your root level, to include the google-services plugin.
Build.gradle
As we are implementing firebase authentication in our android app so we’ll add firebase authentication dependency in a app/build.gradle file and apply plugin line at the bottom of the file to enable the Gradle plugin.
Build.gradle
Now sync the project with gradle.
You can use other features of firebase as per your own requirements by using other dependencies. See the list of available libraries below.
Gradle Dependency Line |
Service |
com.google.firebase:firebase-core:15.0.2 |
Analytics |
com.google.firebase:firebase-database:15.0.0 |
Realtime Database |
com.google.firebase:firebase-firestore:16.0.0 |
Cloud Firestore |
com.google.firebase:firebase-storage:15.0.2 |
Storage |
com.google.firebase:firebase-crash:15.0.2 |
Crash Reporting |
com.google.firebase:firebase-auth:15.1.0 |
Authentication |
com.google.firebase:firebase-messaging:15.0.2 |
Cloud Messaging |
com.google.firebase:firebase-config:15.0.2 |
Remote Config |
com.google.firebase:firebase-invites:15.0.1 |
Invites and Dynamic Links |
com.google.firebase:firebase-ads:15.0.0 |
AdMob |
com.google.firebase:firebase-appindexing:15.0.0 |
App Indexing |
com.google.firebase:firebase-perf:15.2.0 |
Performance Monitoring |
com.google.firebase:firebase-functions:15.0.0 |
Cloud Functions for Firebase Client SDK |
com.google.firebase:firebase-ml-vision:15.0.0 |
ML Kit (Vision) |
com.google.firebase:firebase-ml-tensorflow:15.0.0 |
ML Kit (Custom Model) |
2.IntegrateFirebase using firebase Assistant:
Open Android Studio project in which you want to add firebase
Click Tools->Firebase then click to expand the listed features in a Firebase Assistant
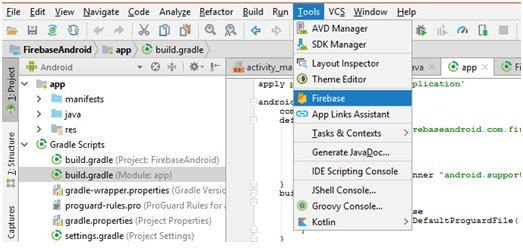
Select the type of firebase feature you want to use in your app.We are using firebase auth so we have clicked Authentication.
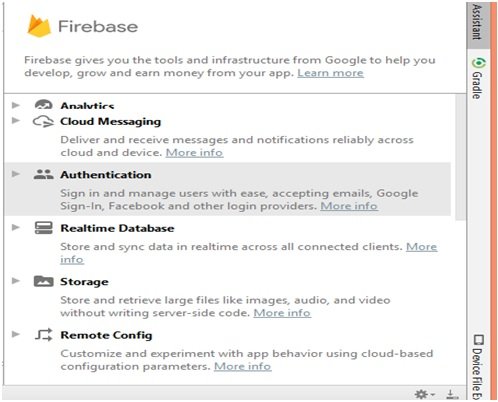
Click the Connect to Firebase button to connect to Firebase and add the necessary code to your app, and if you have already registered your project on a Firebase console then click on “Add Firebase Authentication to your App”.
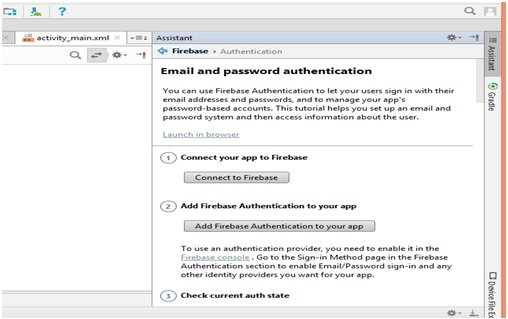
Click on “Accept Changes” button to add the firebase-core library which helps you to add authentication dependencies to your application.
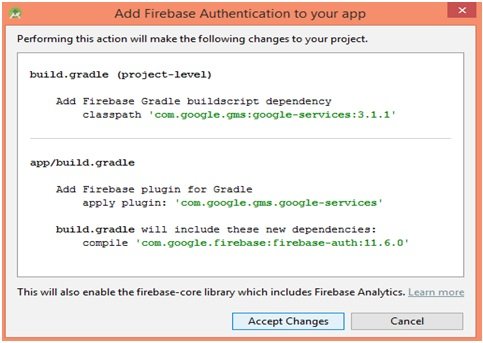
Register User with using Firebase authentication
Click on a link to visit firebase panel https://firebase.google.com/
Click on “Authentication” on the left side of the firebase panel.
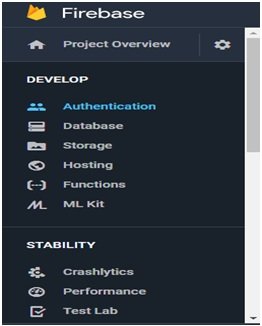
Click on “Set Up Sign In Method” button.
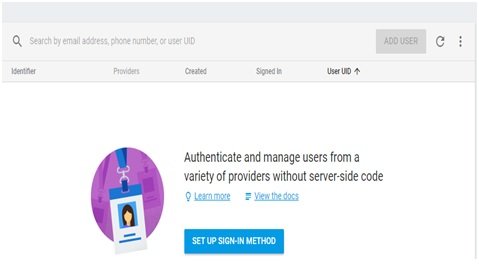
Now click onEmail/Password provider , click enable and save it.
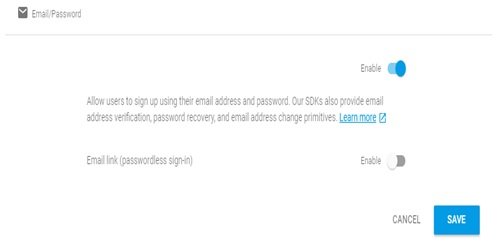
If you would like to integrate the Firebase libraries into one of your own projects, you need to perform a few basic tasks to prepare your Android Studio project.You have already done the integration steps above including dependencies in build.gradlefiles , now come to project implementation.
Android Project Implementation:
Now open the android studio’s project you have created.My project was “FirebaseAndroid”.
Open activity_main.xml file from your project res/layout folder, add the below code in that file
activity_main.xml
Add the below code in string.xml file of your project resources folder.
string.xml
Open the MainActivity.java file of your project and add the below code in it. MainActivity.java
I have declared the firebase authentication object in this class
And then initialized the object inside onCreate() method.
Bind all the layout views with java file.
Initialized the progress dialog box to use it later in the code.
Once the user_registration() method will be called , it will first check the email and password fields, if they are not empty then will seta message on a dialog box "Registering Please Wait... "by calling a function setMessage() and will start creating a user by calling a method createUserWithEmailAndPassword() with the help of “firebaseAuth_obj”
object of a FirebaseAuthclass. In this method we’ll pass two parameters email and password which are necessary for user registration.
The OnCompleteListener will check the task is completed or not.
You can’t perform network-related operations without the internet permission, so add the following line to your project’s manifest file:
Running Android Application:
Click on a Register button and you’ll get the success message , then go to the firebase console and check the registered user there.
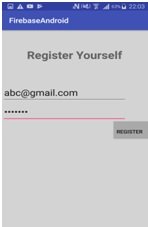
Congratulations,Your registration is done.
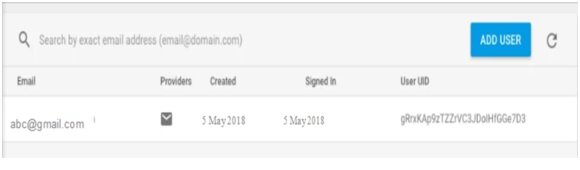