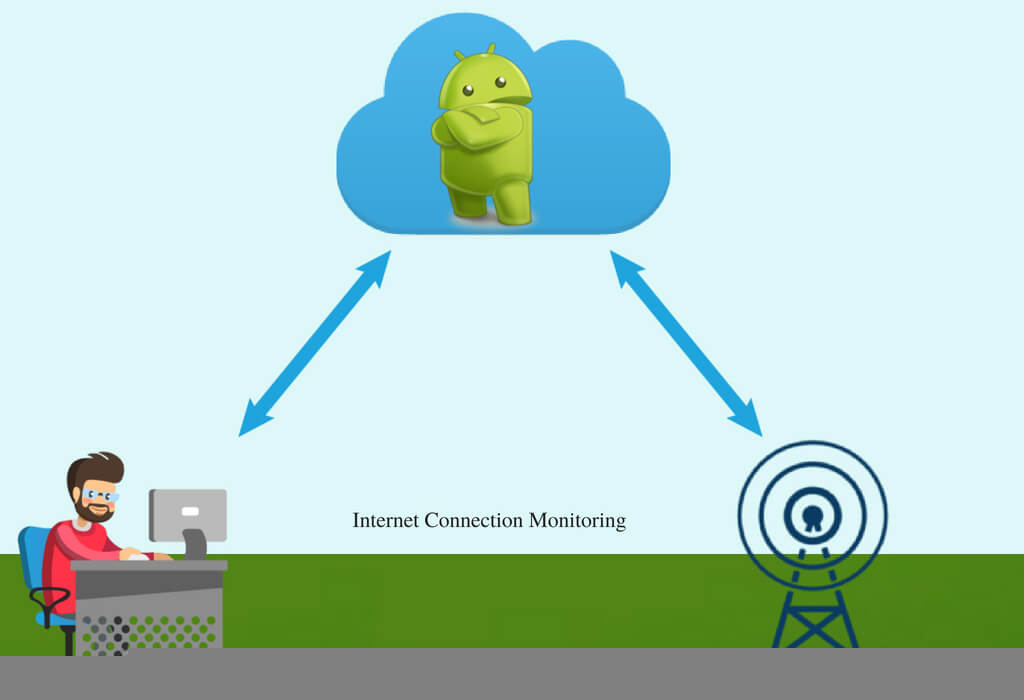
Broadcast receiver is a medium through which communication is done with the registered app and the outside OS system.We needs to register and unregister this functionality according to our requirement. At first we have to register each broadcast receiver to get appropriate notification during desired situations. Broadcast receiver receives notification for a small span of time so we can’t do any kind of long running process over here and we need to unregister the same when app goes to background specifically when app is going to pause mode. It notifies the entire application event that is registered within it.For example, the Android system sends broadcasts when various system events occur, such as when the system boots up or the device starts charging, or battery status or internet connectivity changes, or device received some messages or push notifications. We receive all the notification through receiver.
One important thing is that previously we declare receiver to manifest folder but after API level 25 we need to implement context-registered receiversthat we have discussed later.As we need to retrieve data from server and show it to app it is very important that your app is connected to Internet through mobile data or Wi-Fi and connection condition is good enough to fetch the data from server to App so that user can perform their desired functions. For this we need to check internet connection at every point of time when user request for server data.
It is very easy method to check all the time internet connectivity through Broadcast receiver. It will notify at any point of time when network connection is discontinued or network status changed.In this blog Android Application Development Services providerwill discuss about internet connectivity detection through BroadcastReceiver.
Purpose:Information about Internet connection status through Broadcast Receiver in Android.
Scope
IDE: Android Studio.
Procedure:
Step 1:
At first in your android.Manifest file enable internet, access network state permission and register connectivity changeactionfor Broadcast Receiver. Following are codes:
Above we have defined connectivity change intent. So it will notify easily when network connection is changed (Wi-Fi or mobile data) and after that broadcast receiver will be called for notification.
Step 2:
Next we need to create a class ConnectivityDetection which will extend BroadcastReceiver. This is a receiver class which will be notified whenever there is change in network or internet connection.We need to check onReceive() method. This method will be called every time as network changes. Here we have created a Toast message and displaying current network state. You can compose your custom code in here to handle changes in connection state.
Remember that for Wi-Fi result run it on real device. Otherwise it may not detect properly in emulator.
Each time you implement a BroadcastReceiver, you need to register it in the onResume method of your app and unregister it in the onPause method, as follows:
Now we need to implement Networkstatus class. Below we have detected Wi-Fi, Mobile and not connected status. We have assigned a numeric number for every status change. Following are codes: